Hey, Adela! Can you write me a function? Called circle_circumference
. You send it the radius of a circle, and it returns its circumference.
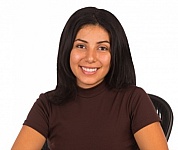
Adela
OK. So, the name is circle_circumference
, it takes one parameter. Here's the first bit.
- def circle_circumference(radius):
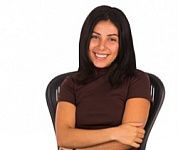
Adela
Hmm... I'll add the return
, to remind myself the function sends something back.
- def circle_circumference(radius):
- # Something here
- return circumference
Note
The name, parameters, and return type of a function are called the function's signature.
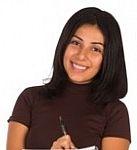
Adela
Now the missing bit.
- def circle_circumference(radius):
- circumference = 2 * radius * 3.14159
- return circumference
Testing
How would you test the code?
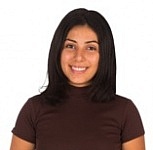
Adela
I'd work out some circumferences I know are correct, and compare them with what my function computed.
Let's see... well, our googly friend can help.
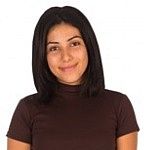
Adela
I'll add some test code...
- def circle_circumference(radius):
- circumference = 2 * radius * 3.14159
- return circumference
- print(circle_circumference(5))
What should the program print?
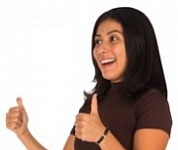
Adela
It works, buckaroos! The program says 31.4159
.
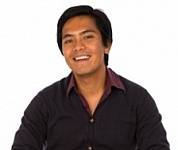
Ray
It's a little off, though, isn't it? Your code said 31.4159
, but Google said 31.41593
.
That's a rounding error, and is normal with floats.
If we want the program to be more accurate, we could use a more accurate value for π (pi). Python has one in the math
library.
- import math
- def circle_circumference(radius):
- circumference = 2 * radius * math.pi
- return circumference
- print(circle_circumference(5))
What's the output now?
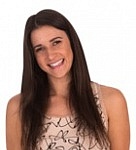
Georgina
I got 31.41592653589793
. That's a tiny difference, compared to 31.4159
. About a ten thousandth of a %. Ish.
Aye. If we were planning to send goats to Mars, it might be important. For most purposes, it doesn't matter.
Type in a function called rect_area
. It computes the area of a rectangle, given two parameters: width and length.
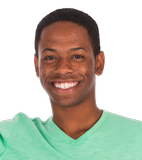
Ethan
Here's what I got.
- def rect_area(width, length):
- area = width * length
- return area
- area = rect_area(5, 6)
- print('Area:' + str(area))
Good!
Let's practice some more.