Log in
If you're a student, please log in, so you can get the most from this page.
String expressions again
Earlier, you did some string expressions, like first_name + last_name
. A string expression is some calculation that gives you a string result. You can put the result into a variable...
- full_name = first_name + last_name
... output it...
- print(first_name + last_name)
So we have:
- String constants, like "There once was a ship that put to sea, ".
- String variables, like
ship_name
. - String expressions, like
'The name of the ship was ' + ship_name
.
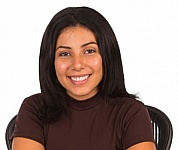
Adela
Hey! I just noticed something. You can't look at a variable name, and tell whether it contains a string or a number.
Yes, you're right. It depends on how the variable is used. Here's some valid code:
- ship_name = "Billy o' Tea"
- first = "There once was a ship that put to sea,\n"
- second = "And the name of the ship was the " + ship_name + ',\n'
- final = first + second
- print(final)
What does that \n
thing do in Python? You might need to look that one up.
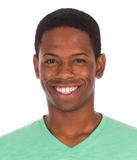
Ethan
It lets you type the Enter key in a string.
Right! Want a g in your string, you type g. Want a z in your string, you type z. Want an Enter in your string, you type \n.
Run that code, and see what you get.
Here's what the Variable Explorer showed me:
All four variables are strings.
Numeric expressions
A numeric expression gives you a numeric value, like meal_cost * 0.15
.
We have:
- Numeric constants, like 42.
- Numeric variables, like
the_ultimate_answer
- Numeric expressions, like
meal_cost * 0.15
Here's some code:
- first = 2.4
- second = 2.2
- final = first + second
- print(final)
Here's the Variable Explorer:
This time, first
, second
and final
are all numbers, not strings.
Both programs have this line:
- final = first + second
+
means something different in each program. In the first, it means "squoosh strings together." In the second, it means "add two numbers."
Numeric operators
The most common are:
Operator | Meaning |
---|---|
** | Raise to a power, e.g., 5**2 is 25 |
* | Multiply |
/ | Divide |
+ | Add |
- | Subtract |
As with regular arithmetic, operators have a precedence. Take this:
- llama = 2 + 3**2 - 6/2 + 5*2 - 2**3
First, Python does the **
, because it has the highest precedence. Python scans left to right, doing them in order.
- llama = 2 + 9 - 6/2 + 5*2 - 2**3
Then the second **
:
- llama = 2 + 9 - 6/2 + 5*2 - 8
Next, Python does the *
and /
. They have equal priority, and are done left to right.
- llama = 2 + 9 - 3 + 5*2 - 8
Then:
- llama = 2 + 9 - 3 + 10 - 8
Now the +
and -
. They have equal priority, and are done left to right.
- llama = 11 - 3 + 10 - 8
- llama = 8 + 10 - 8
- llama = 18 - 8
- llama = 10
So, Python's built-in order is...
**
, left to right, then...*
and/
, left to right, then...+
and-
, left to right.
Another one:
- cat = 3 - 5 + 2 * 2 ** 2 + 3
... would be done in this order...
- 3 - 5 + 2 * 2 ** 2 + 3 becomes 3 - 5 + 2 * 4 + 3
- 3 - 5 + 2 * 4 + 3 becomes 3 - 5 + 8 + 3
- 3 - 5 + 8 + 3 becomes -2 + 8 + 3
- -2 + 8 + 3 becomes 6 + 3
- 6 + 3 becomes 9
Parentheses
You can use parentheses to change the order of operations. For example:
- llama = (2 + 3)**2 - 6/2 + (5*2 - 2)**3
2 + 3
would be done first, then 5*2 - 2
(*
first and then the -
). The result is 534.
Rounding
Say you wanted to give someone a 5% discount on an item costing $2.95. A simple calculation:
- final_price = 2.95 * 0.95
- print('Final price: ' + final_price)
What's the result?
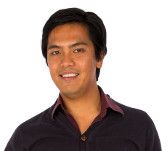
Ray
It's 2.8025. So that's $2.80 plus a quarter of a cent.
Right. You wouldn't charge the quarter cent. Instead, you'd round the output value. The round
function does that.
- final_price = 2.95 * 0.95
- print('Final price:' + str(round(final_price, 2)))
This one rounds to at most two decimal places.
It's better to round as late as possible in your code, just during output. That's what the code above does; round
is in the print
statement.
If you round earlier, you risk losing precision for later calculations.
Notice the code above doesn't change the value of final_price
to 2.80. Only the display is affected. That's the right way to do it.
Run the code, and you'll get 2.8
, not 2.80
. You can force the missing 0
, but let's not worry about it.
Your turn
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Exercise
Quadratic roots
Write a Python program to compute the roots of a quadratic equation. Here's one, let's call it Candice:
Solve it with this formula. We'll name it Billy:
Candice has two solutions. Billy gives both. See that +/- thing? For one solution, it's +. For the other, it's -. So, use Billy twice, once for each solution.
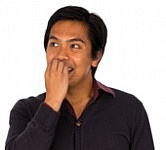
Ray
I don't remember much algebra.
You don't need to. Just get the inputs, and use the formula. You know all you need.
Here's some I/O:
- a? 1
- b? 6
- c? 8
- First solution: -2.0
- Second solution: -4.0
For the square root, put this at the top of your code:
- import math
Then you can compute square roots like this:
- result = math.sqrt(something_goes_here)
Many quadratics don't have real roots, and will crash your program. Here's an example using 1 for a, b, and c:
This is normal. Check the formula again, the bit under the square root:
If 4*a*c is more than b*b, you'll get a negative value. For example, when a, b, and c are all 1 (as in the screenshot), b*b - 4*a*c = 1*1 - 4*1*1 = -3.
Trying to find the square root of a negative value is a no-no in standard algebra. It does work when you use complex numbers, where the constant i is the square root of -1, but we're not going there.
Python's standard float type doesn't know what to do with the square root of a negative, and it crashes. That's OK, and expected.
To test your code, use values for a, b, and c that have real solutions. There's a useful page that lets you try different values for a, b, and c. Your program should produce the same results as the program on that page, except when 4*a*c is more than b*b. Here's what that page says when a, b, and c are all 1:
The roots are complex, so the values should crash your program.
However:
Your program should produce the same roots, -4 and -2.
You'll learn a lot about data validation later in the course. You could use what you'll learn to avoid crashy crashy. But let's not worry about it for now.
Include comments for the IPO chunks.
Upload a zip of your project folder. The usual coding standards apply.