Tina's Louie
Here's the program again, without the syntax error we fixed.
- first_name = input('What is your first name? ')
- last_name = input('What is your last name? ')
- full_name = first_name + last_name
- print('Hello!')
- print('My name is')
- print(full_name)
Run it, and the first line will ask you a question.
- What is your first name? Blovian
What happens to the data you type? It goes into a variable.
Variables
A variable is a chunk of computer memory with a name. Knowing how to use variables is a Very Important Thing. Much of programming is putting data in and getting data from variables.
When Python runs this line...
first_name = input('What is your first name? ')
... the first thing it does is notice you've referred to a new variable, first_name
. Python grabs some memory, and gives it the name first_name
.
Next, it sees the =
. The =
is the assignment operator. It takes data from the right-hand side of the =
, and puts it into the variable named on the left-hand side. For example:
legs = 4
This tells Python:
- If there's no
legs
variable already, create it in memory. - Put 4 in it.
If I'd been designing the language, I'd have used an arrow instead:
legs <- 4
<-
more clearly shows that whatever is on the right goes into the variable on the left. But we're stuck with =
as the assignment operator.
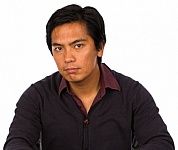
Ray
I don't get it. The code is...
first_name = input('What is your first name? ' )
Shouldn't "What is your first name? " go into first_name
?
No, "What is your first name? " is a prompt
, a question for the user. The input
function shows the prompt, and waits. Whatever the user types in the console is what input
returns. So here...
Ray
goes into first_name
.
The console remembers variables in programs you run. So, I can ask the console about first_name
.
The thing on the right doesn't have to be a fixed value. For example:
sales_tax = price * 0.06
This tells Python to:
- If it doesn't already exist, make a variable called
sales_tax
in memory. - Take whatever value is in the variable
price
, multiply it by 0.06, and put the result intosales_tax
.
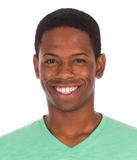
Ethan
What happens if there isn't a variable called price
?
Good question! Python won't create price
. It only creates variables named on the left of the =
. If price
doesn't exist, Python won't know what value to multiply by 0.06.
The program gives you an error message, and stops. In other words, it crashes. The error message won't be something friendly, like "Sorry, I need a price." I'll be something computery that'll make regular people nervous.
In sales_tax = price * 0.06
, *
means multiply. There's +
, -
, /
(means divide), and other things.
BTW, fixed values, like 4 and 0.06, are called constants because they never change. 4 is always 4, no matter how many times you run the program. 0.06 is, well, 0.06. It can't change. It's constant.
Yet another example:
total = price + sales_tax
Python...
- If the variable
total
doesn't exist, make it. Take a little piece of memory, and name ittotal
. - Take what's on the right (whatever's in
price
plus whatever's insales_tax
), and put it in the variabletotal
.
You can put anything that returns a value on the right.
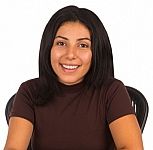
Adela
Do price
and sales_tax
change when you access them?
No, only the variable on the left of =
, total
, changes. Taking data from a variable to do a calculation doesn't change it.
Naming variables
Here are some good variable names:
first_name
total_legs
best_doggo_name
score
sales_tax
A few things. First, they're all lowercase. Unfortunately, Python variable names are case-sensitive. So total
and Total
are different variables. Try this in the console:
- total = 6
- print(Total)
It's easy if we use lowercase for all variable names. Let's make that a rule for ourselves.
Variable naming rule
Make all variable names lowercase.
Try this in the console:
- print = 7
- print(print)
The first line works, kinda, but blocks the print()
function. So, another rule: don't use Python keywords as variable names.
Variable naming rules
- Make all variable names lowercase.
- Don't use Python keywords as variable names.
The third rule. sales_tax
is a two-word variable name. Makes sense. We could use salestax
, but it's not as easy to understand.
What about sales-tax
instead of sales_tax
? Would that OK?
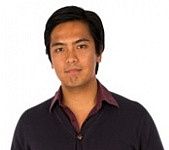
Ray
Er, I just tried it in the console:
- sales-tax = 4
- *** SyntaxError: cannot assign to operator
Sometimes Python's error messages don't tell you what the error actually is. Anyone know what's wrong?
What's wrong with Ray's code?
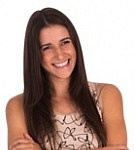
Georgina
I think I know. Python thought the - in sales-tax
meant minus, like sales
minus tax
.
Indeed. Computers are stupid. They have no common sense at all. You have to allow for that.
Variable naming rules
- Make all variable names lowercase.
- Don't use Python keywords as variable names.
- Use underscores (
_
) to separate parts of variable names.
Last, the most important rule of all!. What's the business purpose of this code?
- v2 = v1 * v3
- v0 = v1 + v2
What's the business purpose of the code?
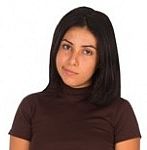
Adela
No way to tell. Could be anything.
Aye. How about this?
- sales_tax = price * sales_tax_rate
- total_price = price + sales_tax
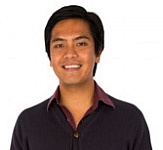
Ray
That's easy to follow.
Yes. So:
Variable naming rules
- Make all variable names lowercase.
- Don't use Python keywords as variable names.
- Use underscores (
_
) to separate parts of variable names. - Use meaningful variable names.
Follow these rules in all your programs.
If you forget what they are, there's a page called Code standards in the Extras section:
String constants
Here's our line again:
first_name = input('What is your first name? ')
Remember, the question is a called a prompt, because it prompts the user to do something.
input
is a function. (You'll learn more about functions later.) It's a bit o' code someone wrote. It shows the prompt in the console, and waits for you to type something.
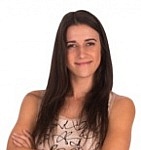
Georgina
Is the prompt a variable, too?
Good question. No, it isn't. Remember earlier we said what a value that doesn't change is? Like 4.
What do you call a value like 4 or 3.14159, that doesn't change?
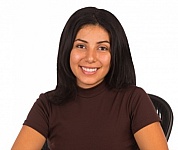
Adela
That's a constant, isn't it?
Right! 4 and 3.14159 are numeric constants. In this code...
- print(4)
-
... you always get 4, not matter how many times you run the program.
'What is your first name? '
is a string constant. A string is a buncha characters, anything you can type on the keyboard.
A string constant doesn't change. All users see the same question every time (though their answers will be different). Python uses quotes, either single ('
) or double ("
) to mark string constants.
This program...
- print('Goats are cool!')
-
... always outputs the same thing.
Here's our line again:
first_name = input('What is your first name? ')
To run the statement, Python...
- If
first_name
doesn't exist, take some memory and name itfirst_name
. - Show
What is your first name?
in the console. - Wait until the user types something and presses Enter.
- Put whatever they typed into the variable
first_name
.
Here's what I typed:
So Kieran went into first_name
.
Expressions
OK, now line 3:
full_name = first_name + last_name
Like the first two lines, it works out a value on the right, and puts it into the variable named on the left.
first_name + last_name
is an expression, a calculation of some sort. You'll use a lot of them in the course.
If I type Kieran for the first name, and Mathieson for the last name, what's the value of full_name
? Hint: for strings, +
means put two strings together.
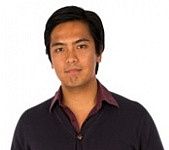
Ray
first_name
is Kieran, last_name
is Mathieson, so full_name
would be Kieran Mathieson.
Makes sense, but it's not quite right. Here's some console action:
- What is your first name? Kieran
- What is your last name? Mathieson
- Hello!
- My name is
- KieranMathieson
No space between the first and last names.
Why didn't the program add a space between the first and last names?
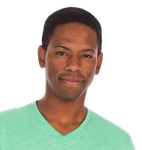
Ethan
Maybe... because we didn't tell it to?
Right!
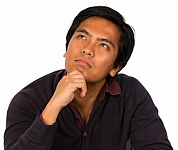
Ray
But the variables are called first_name
, last_name
, and full_name
. It's obvious what we want.
Obvious to you and me, but not to the computer. Here's an important thing to remember:
Computers don't know what you intend to do, only what you tell them to do.
Computers have no common sense. They're stupid machines. Only the programs we write make them smart.
BTW, the names of the variables don't matter to the computer. This code...
- x = input('What is your first name? ')
- y = input('What is your last name? ')
- z = x + y
... would do the same thing.
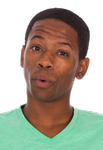
Ethan
Wait. z = x + y
could mean anything. Like, adding tax to a price, or... well, anything!
Yes. Computers don't care what variables are named, but humans do. It's easier to understand programs when variables have meaningful names.
Output
Here's the program again:
- first_name = input('What is your first name? ')
- last_name = input('What is your last name? ')
- full_name = first_name + last_name
- print('Hello!')
- print('My name is')
- print(full_name)
print
outputs a line. It can show constants, like print('Hello!')
, variables, like print(full_name)
, or expressions, like...
- print(price * (1 + tax_rate))
String constants have quotes around them. So 'What is your first name?'
is a string constant. The quotes mark the beginning and end of a string.
What would this code output?
- first_name = 'Sindhu'
- last_name = 'Vee'
- full_name = 'first_name + last_name'
- print(full_name)
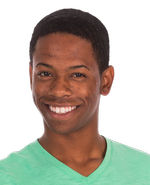
Ethan
You're being tricky here, Kieran. Line 3 has quotes in it. So... I'm guessing the output will be...
first_name + last_name
But I'm not sure.
Yes! You got it. You can run the code in Spyder, and see what you get.
' and " create a string constant. 'first_name + last_name'
is a string constant, and will never change, no matter what you type for first and last name.
In our name tag program, we have:
- full_name = first_name + last_name
- print(full_name)
No quotes. Python takes the value of the variable first_name
, appends the value of the variable last_name
, and puts the result into full_name
.
Adding a space
We still have a problem. There's a missing space in full_name
:
- What is your first name? Kieran
- What is your last name? Mathieson
- Hello!
- My name is
- KieranMathieson
The problem is this line:
- full_name = first_name + last_name
We need to change the expression to get a space between the first and last names. How?
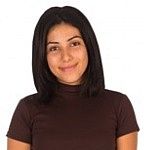
Adela
Just type it in. Put another space after the +
.
Makes sense. Give it a try.
Did Adela's solution work?
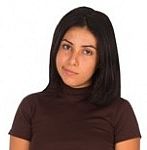
Adela
Well, 💩. The output didn't change.
Right. When Python reads your code, it ignores extra spaces that aren't between quotes.
So these do the same thing:
Spaces in quotes? They show. Spaces in the rest of the code? Irrelevant. (Unless they're indenting, which we'll cover later. Forggedaboudit for now.)
We need to tell Python we want one space between the names.
How would you add the space?
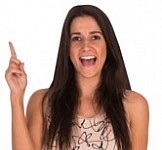
Georgina
Ooo! Idea! We have console output like...
Python does add spaces there. If we can figure out why, we can do the same thing for the name.
Yes! You're on the right track!
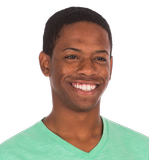
Ethan
The spaces are in quotes with the rest of the text:
first_name = input('What is your first name? ')
So, what if we got rid of everything in the prompt except one space? Like...
' '
Put that between the names? Just a space in quotes.
Woohoo! That's it! But how do you get ' '
between the names?
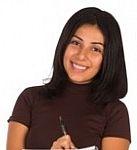
Adela
Hey! We joined strings together with +
:
full_name = first_name + last_name
Instead of first name and then last name, we want first name, then a space, then last name. Could we use another +
? Between the names?
Aye!
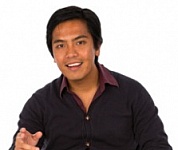
Ray
Like:
full_name = first_name + ' ' + last_name
YES, THAT'S IT!
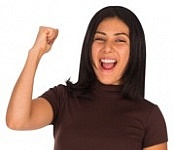
Adela
I'm gonna add it to the program...
It works!
You guys rock!
A space is just another character you can type on the keyboard. If you want spaces in your output, you need to tell Python to put them in.
BTW, ' '
is not the same as ''
(that's two quotes with nothing between them). The second is an MT (empty) string. It has no characters at all.
Blank lines
Here's some code:
- print('Doggos rock!')
- print('Cattos are OK')
That will output:
- Doggos rock!
- Cattos are OK
What if you wanted a blank line between them?
- Doggos rock!
- Cattos are OK
An easy way is to add another print, with no content:
- print('Doggos rock!')
- print()
- print('Cattos are OK')
That will give you:
- Doggos rock!
- Cattos are OK
Summary
Here's the main points. Reading them again helps link memories together, making your study time more effective.
- A variable is a small piece of memory with a name.
=
is the assignment operator, as inhooves = legs * 4
. The value on the right is put into the variable on the left.4
and31.3
are numeric constants.'Oakland wins the game!'
and'Doggos are the best things ever!'
are string constants.- Use the
+
operator to join strings together.+
does other things, too, but with strings, it appends them. - Computers don't know what you intend to do, only what you tell them to do.
- If you want spaces in the output, you need to add them somewhere inside quotes.
- This will add a blank line: print()
Exercises
Fave animal
Write a program that asks the user questions about their fave animal, and outputs a message about it. Here's some I/O:
- Fave animal
- ===========
- What is the genus of your fave animal? canis
- What is the species of your fave animal? lupus
- What is the subspecies of your fave animal? familiaris
- What is the common name of your fave animal? dog
- - - - - - - - - - - - -
- Your fave animal is canis lupus familiaris, better known as the dog.
(The line of dashes is part of the output, as is the following line starting with "Your fave...". Your program should print them.)
User input is highlighted. The rest is output by your program.
Users can input any animal deets they want.
Format your I/O exactly as is given, with underlines, blank lines, etc.
Hint: This will print a blank line: print()
Upload a zip file of your project folder.