Hey, Ray! Wanna do some coding?
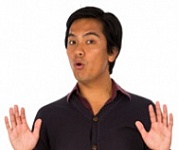
Ray
Who, me?
The others are better at this.
Maybe, maybe not. Watching you work will help the people reading this learn from you.
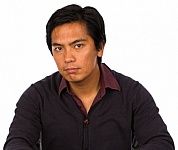
Ray
Wait. What people reading this? What do you mean?
Never mind. It's a fourth-wall thing.
Anyway, write a program to work out whether a goat is allowed on the Goatercoaster.
Ol' Zeke explains.
- - CUT SCREEN HERE - -
Well, youngun, old goats like me, older than eight, tell 'em they's too old. Ol' goats suck, anyways. Ol' humans are worse. Smell funny, can't use a phone right, don't know the right bands. They's no good.
Oh, but maybe ask 'em they's name, and, like, personalize the rejection.
Middle-aged goats, between four and eight, tell 'em: "Welcome aboard!" With they's name, too.
Young adult goat, age two to four, tell 'em to have fun on the Goatercoaster.
Younger than that, tell 'em the Goatercoaster is too scary for kids.
- - CUT SCREEN HERE - -
Here's some I/O:
- What's your name? Bluus
- How old are you? 9
- Sorry, Bluus, you're too old.
Or this:
- What's your name? Joon
- How old are you? 5.5
- Welcome aboard, Joon!
More:
- What's your name? Klunu
- How old are you? 3.5
- Have fun on the Goatercoaster, Klunu!
Some more:
- What's your name? Destroyer of Gates
- How old are you? 1
- Sorry, Destroyer of Gates, the Goatercoaster is too scary for kids.
You can assume users never make typing mistakes. Unrealistic, and we'll handle that later.
Oh, I'll just leave this here.
GO!
Not graded. So why do it?
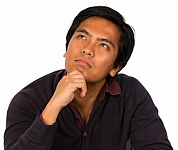
Ray
I don't know anything about Goatercoasters. Don't panic. Look for patterns underneath.
Let's check the I/O...
- What's your name? Klunu
- How old are you? 3.5
- Have fun on the Goatercoaster, Klunu!
Maybe it's an IPO? There's input, and output. Maybe processing to choose the message?
Is that right? Adela?
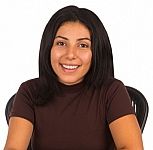
Adela
Yes, you got this.
Note
Ray was nervous when he looked at the surface of the task, since Goatercoasters are new to him. But this task isn't about Goatercoasters, not really. When Ray looked under the surface, he found a pattern he has used before.
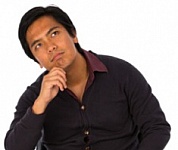
Ray
OK, I'll write out comments for the pattern.
- # Input.
- # Processing.
- # Output.
Note
A good start, putting in comments for the big chunks of the program.
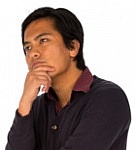
Ray
Now what? Umm... let's look at first part, the input.
What's the I/O again?
- What's your name? Klunu
- How old are you? 3.5
- Have fun on the Goatercoaster, Klunu!
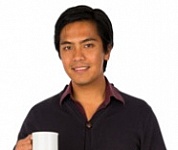
Ray
So there's name, and age. I'll add some more comments.
- # Input.
- # Get name.
- # Get age.
- # Processing.
- # Output.
Note
Ray is writing the program in tiny steps. That's the right way to do it.
Not graded. So why do it?
Not graded. So why do it?
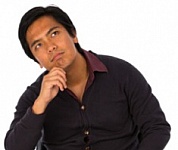
Ray
Let's see...
- # Get name.
- goat_name = input("What's your name? ")
- # Get age.
- goat_age = input('How old are you? ')
Ray made a mistake here. What is it?
Let's see if Ray finds the problem.
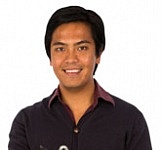
Ray
I'll test this in Spyder so far...
- What's your name? Klunu
- How old are you? 3.5
Test code as you write it. Write a bit, test a bit, write a bit, test a bit.
It's more efficient than writing an entire program at once, and then testing it.
Note
Testing so far hasn't shown any issues.
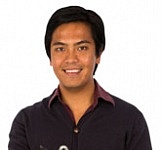
Ray
OK, processing. Looking at what Zeke said...
- - CUT SCREEN HERE - -
Well, youngun, old goats like me, older than eight, tell 'em they's too old. Ol' goats suck, anyways. Ol' humans are worse. Smell funny, can't use a phone right, don't know the right bands. They's no good.
Oh, but maybe ask 'em they's name, and, like, personalize the rejection.
Middle-aged goats, between four and eight, tell 'em: "Welcome aboard!" With they's name, too.
Young adult goat, age two to four, tell 'em to have fun on the Goatercoaster.
Younger than that, tell 'em the Goatercoaster is too scary for kids.
- - CUT SCREEN HERE - -
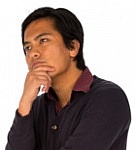
Ray
Where to start? Hmm, a lot depends on the goat's age. Let's start there.
Old goats don't get to ride.
- # Processing.
- if goat_age > 8:
- print "Sorry, you're too old."
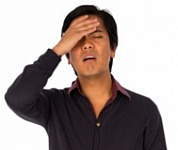
Ray
OK, let me test it again... What the hell?
- What's your name? Harlowe
- How old are you? 9
- ...
- TypeError: '>' not supported between instances of 'str' and 'int'
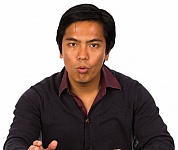
Ray
OK. Ray, it's OK. Don't panic.
Let's google the error message. Python TypeError not supported instances of str and int... OK, here's a page that might help. It says, "The comparison operator cannot be used to compare strings and numbers."
Hmm. Comparison operator is the >. Compare strings and numbers. I'm not sure what that means...
OK, I'll check the Variable Explorer.
Let's see... I typed 9 for age, there it is... wait, the type is string? 9 is a number. Why does...
Oh! I remember now! Let me check stuff from earlier... There it is! input()
always returns a string. I need to convert it to a number.
Let's try this.
- # Get age.
- goat_age = float(input('How old are you? '))
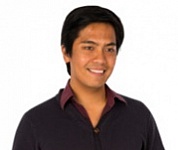
Ray
Yay! No errors!
- # Input
- # Get name.
- goat_name = input("What's your name? ")
- # Get age.
- goat_age = float(input('How old are you? '))
- # Processing.
- if goat_age > 8:
- print "Sorry, you're too old."
OK. The I/O when I run it is...
- What's your name? Torrent
- How old are you? 9
- Sorry, you're too old.
Is the I/O correct so far?
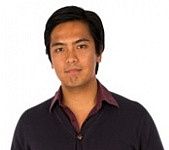
Ray
Oh, wait a minute... Let me check the sample I/O again.
- What's your name? Bluus
- How old are you? 9
- Sorry, Bluus, you're too old.
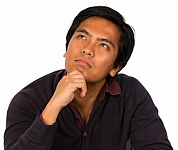
Ray
The name should be in there.
- # Get name.
- goat_name = input("What's your name? ")
- # Get age.
- goat_age = float(input('How old are you? '))
- # Processing.
- if goat_age > 8:
- print("Sorry," + goat_name + ", you're too old.")
Will that produce the right output?
I/O:
- What's your name? Hattie
- How old are you? 9
- Sorry,Hattie, you're too old.
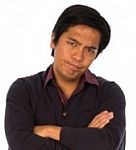
Ray
💩! The spacing isn't right.
I just need to add another space before the name, after the ,.
- # Input.
- # Get name.
- goat_name = input("What's your name? ")
- # Get age.
- goat_age = float(input('How old are you? '))
- # Processing.
- if goat_age > 8:
- message = "Sorry, " + goat_name + ", you're too old."
OK, I'll run that, and check out the VE (variable explorer).
Yay!
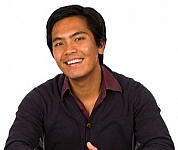
Ray
Now for the next bit.
- # Processing.
- if goat_age > 8:
- message = "Sorry, " + goat_name + ", you're too old."
- elif goat_name < 8 and goat_age > 4:
- message = 'Welcome aboard, ' + goat_name + '!'
See any issues?
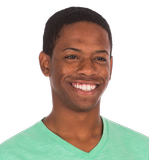
Ethan
Hey, Ray! That rhymes!
What if the age is 8?
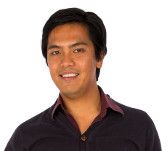
Ray
Huh?
Oh! I see what you mean. That would slip through the cracks.
- # Processing.
- if goat_age > 8:
- message = "Sorry, " + goat_name + ", you're too old."
- elif goat_name <= 8 and goat_age > 4:
- message = 'Welcome aboard, ' + goat_name + '!'
Can the second if
be simpler?
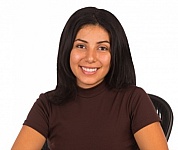
Adela
By the time you get to the second if
, you know age is less than or equal to eight. If age was more than 8, the first if
would already have taken care of that. So, do you need the first part of the if
test?
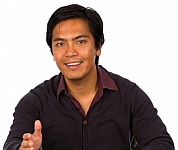
Ray
Oh! You're right, Adela! Thanks!
- # Processing.
- if goat_age > 8:
- message = "Sorry, " + goat_name + ", you're too old."
- elif goat_age > 4:
- message = 'Welcome aboard, ' + goat_name + '!'
Woohoo!
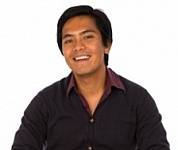
Ray
Time to finish up.
- # Input.
- # Get name.
- goat_name = input("What's your name? ")
- # Get age.
- goat_age = float(input('How old are you? '))
- # Processing.
- if goat_age > 8:
- message = "Sorry, " + goat_name + ", you're too old."
- elif goat_age > 4:
- message = 'Welcome aboard, ' + goat_name + '!'
- elif goat_age > 2:
- message = 'Have fun on the Goatercoaster, ' + goat_name + '!'
- else:
- message = 'Sorry, ' + goat_name + ', the Goatercoaster is too scary for kids.'
- # Output.
- print(message)
Test the code. Does it work?
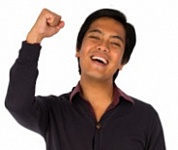
Ray
It works! Victory or Sovngarde!
Skyrim forever!
Summary
- Look under the surface of the task for patterns you've used before.
- Incremental testing is good.
- The Variable Explorer is good.
input
always returns a string. Remember to convert it to an int, float, or whatever you need.