Matthew's Maggie
Patterns
Tasks are easier if you know some patterns. A pattern is a common way of doing things.
In writing, a common pattern for essays is:
- Introduction
- Body
- Conclusion
- References
Over the years, people have found this a useful structure. Essays about dogs, cars, learning, and dating can all use this structure, even though the topics are different.
There are patterns for writing business letters. This one is a letter template in Writer, a free Word alternative.
There are places for addresses, greeting, signature, and so on. No matter what a business letter is about, you can use this pattern.
Programming patterns
There are programming patterns, too. Common ways of doing things. Sometimes tasks appear different on the surface, but their solutions use the same patterns.
OK, I'm gonna say that again. It's important.
Remember me!
Sometimes tasks appear different on the surface, but their solutions use the same patterns.
Take mad libs, for example. A mad lib is a game where you ask people for words, and fill in a template they haven't seen. Here's one, from Wikipedia:
You ask someone for an exclamation, adverb, etc., fill in the slots, and show them the result. Maybe:
Say we want to write a program for that.
Here's I/O for the name tag program:
- What is your first name?Kieran
- What is your last name?Mathieson
- Hello!
- My name is
- Kieran Mathieson
-
Here's I/O for a mad lib program:
- Please enter an exclamation: Ouch
- Please enter an adverb: stupidly
- Please enter a noun: cat
- Please enter an adjective: brave
- --------------------
- "Ouch!" he said stupidly as he jumped into his
- convertible cat and drove off with his brave wife.
-
What do the I/O of the name tag and mad lib programs have in common?
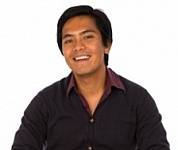
Ray
There's some input first, then some output.
Right. The things being input and output are different in each program. One has first name and last name, the other has nouns, adverbs, etc. But they have a similar input-output sequence.
When two sets of I/O have the same structure, that's a hint the programs behind both sets of I/O might have the same structure, too. In fact, it turns out the two programs do have the same structure.
Not graded. So why do it?
Code structure
Here's the code of the name tag program:
- first_name = input('What is your first name? ')
- last_name = input('What is your last name? ')
- full_name = first_name + last_name
- print('Hello!')
- print('My name is')
- print(full_name)
What's the structure of the program? "Structure" means chunks of code that belong together, doing something you can give a name to. It's not a precise definition, just something programmers find useful.
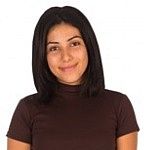
Adela
The first two lines are input. They seem like they belong together.
Good. That's one chunk of code. We have the structure:
- Input
- Other stuff
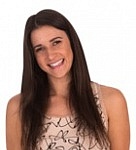
Georgina
How about the last three lines? An output chunk.
Right.
- Input
- Other stuff
- Output
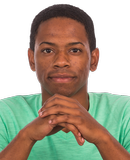
Ethan
What about line 3: full_name = first_name + last_name
It's not input, and it's not output. It takes input, and works out something output needs. I don't know what to call it.
A chunk like that is called processing. Sort of a generic name. You're right that processing takes input data, and does something to it to make data the output needs.
Here's the program's structure:
IPO pattern
Put the chunks together, and you have IPO (input, processing, output), one of the most common programming patterns.
One of the simplest patterns:
- Code to put valid input data into variables.
- Code to work out anything output needs that isn't in the input.
- Code to output variable values.
We'll add more patterns throughout the book. If you're working on an exercise and are not sure how to do it, check out the pattern catalog for ideas.
The output of the name tag program and the mad lib program are similar. So, if IPO works for the name tag program, there's a good chance you can use IPO for the mad lib program.
In fact, IPO does work well for the mad lib program. Knowing the program's structure before you write it makes life easier.
Note
When you start a new task, think about which patterns might be useful.
Comments
You can use comments to show the structure of a program, like this:
- # Input
- first_name = input('What is your first name? ')
- last_name = input('What is your last name? ')
- # Processing
- full_name = first_name + last_name
- # Output
- print('Hello!')
- print('My name is')
- print(full_name)
A comment is a note to yourself or to other programmers. Comments begin with #, and are ignored by Python. Here, each code chunk in IPO has a comment showing where it starts.
Actually, I would add another comment at the top, explaining what the program does, who wrote it, and when:
- # Make a name tag.
- # Input is first and last name.
- # Written by Kieran Mathieson, June 11, Year of the Dragon
- # Input
- first_name = input('What is your first name? ')
- last_name = input('What is your last name? ')
- # Processing
- full_name = first_name + last_name
- # Output
- print('Hello!')
- print('My name is')
- print(full_name)
I also put comments on tricky pieces of code inside chunks. For example, I might explain how a complex calculation works.
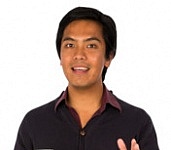
Ray
Why bother with comments? The code is still the same, really.
Say you're a data analyst, and your boss asks you to check out sales data for the south-east region. Two months ago, your colleague Ann wrote a similar program for analyzing international sales. It's usually cheaper to copy Ann's program and change it, rather than write new code from scratch. Cheap is good.
You need to understand Ann's code before you change it. Comments help with that. They also tell you who to call if you need more deets on how the program works.
Summary
Read summaries to reactivate memories for ideas, making them easier to recall.
- Patterns are common ways of doing things.
- When two programs' I/O have the same structure, the programs might have the same structure.
- IPO (input, processing, output) is a common pattern.
- Use comments to show the structure of a program, and to explain tricky code.
- Add comments to the top of the program, explaining what the program does, who wrote it, and when.
- Look under the surface to find a program's structure. You can use that structure for hundreds of tasks.
Exercises
Mad lib
Write a mad lib program. Use any sentence(s) you like, except the ones in the sample below. The user should input at least four words. Use prompts like in this I/O sample:
- Please enter an exclamation: Ouch
- Please enter an adverb: stupidly
- Please enter a noun: cat
- Please enter an adjective: brave
- - - - - - - - - - -
- "Ouch!" he said stupidly as he jumped into his
- convertible cat and drove off with his brave wife.
Zip the folder containing your solution project. The usual programming standards apply. Also, add comments showing the main parts of the program, according to the IPO pattern.