Bonus lessons are optional. If you know HTML or are curious about dynamic webpages, this might interest you.
We had versions of the name tag program that output to the screen, a network printer, or a file. You can also make a webpage from the output. Let's see how.
First, here's a function making the HTML for one name tag.
- def make_html_name_tag(first_name, last_name):
- '''
- Make HTML for one name tag.
- Parameters
- ----------
- first_name : string
- Person's first name.
- last_name : string
- Person's last name.
- Returns
- -------
- name_tag_markup : string
- HTML markup for the tag.
- '''
- name_tag_markup = '<p>Hello!</p>\n'
- name_tag_markup += '<p>My name is</p>\n'
- name_tag_markup += '<p><strong>' + first_name \
- + ' ' + last_name + '</strong></p>\n'
- name_tag_markup += '<hr>\n'
- return name_tag_markup
It puts the HTML into a string variable, and returns it.
Now, let's call the function once for each name tag we want. We'll accumulate the HTML in a string variable.
- # Accumulate name tags HTML.
- tags_html = make_html_name_tag('Wednesday', 'Addams')
- tags_html += make_html_name_tag('Xavier', 'Thorpe')
- tags_html += make_html_name_tag('Tyler', 'Galpin')
- tags_html += make_html_name_tag('Enid', 'Sinclair')
We need to make a complete webpage with a head, body, the usual stuff. This code makes HTML for the entire page in the variable html
. It includes tags_html
, the string we made a moment ago with the markup for all the name tags.
- # Make the page.
- html = '<html>\n'
- html += ' <head>\n'
- html += ' <title>Name tags</title>\n'
- html += ' </head>\n'
- html += ' <body>\n'
- html += ' <h1>Your name tags</h1>'
- html += tags_html
- html += ' </body>\n'
- html += '</html>\n'
Now, we just need to write the HTML to a file.
- # Write HTML to a file
- with open('./name-tags.html', 'w') as name_tags_file:
- # Writing data to a file
- name_tags_file.write(html)
You can download the code and try it.
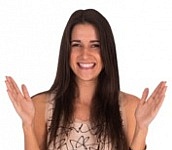
Georgina
That's so cool!
Could you make a page from user input?
Sure. Here's a program for one name tag.
- # Create a webpage with a name tag.
- # Written by Kieran Mathieson, August 30, Year of the Dragon.
- def make_html_name_tag(first_name, last_name):
- '''
- Make HTML for one name tag.
- Parameters
- ----------
- first_name : string
- Person's first name.
- last_name : string
- Person's last name.
- Returns
- -------
- name_tag_markup : string
- HTML markup for the tag.
- '''
- name_tag_markup = '<p>Hello!</p>\n'
- name_tag_markup += '<p>My name is</p>\n'
- name_tag_markup += '<p><strong>' + first_name \
- + ' ' + last_name + '</strong></p>\n'
- name_tag_markup += '<hr>\n'
- return name_tag_markup
- def compute_file_name(first_name, last_name):
- '''
- Compute a file name for the name tag webpage.
- All lowercase, spaces replaced by dashes.
- Parameters
- ----------
- first_name : string
- First name.
- last_name : string
- Last name.
- Returns
- -------
- file_name : string
- File name.
- '''
- file_name = first_name.replace(' ', '-').strip().lower()
- file_name += '-'
- file_name += last_name.replace(' ', '-').strip().lower()
- file_name += '-name-tag.html'
- return file_name
- # Input.
- first_name = input('First name? ').strip()
- last_name = input('Last name? ').strip()
- # Name tags HTML.
- tags_html = make_html_name_tag(first_name, last_name)
- # Make the page.
- html = '<html>\n'
- html += ' <head>\n'
- html += ' <title>Name tag for ' + first_name + ' ' + last_name + '</title>\n'
- html += ' </head>\n'
- html += ' <body>\n'
- html += ' <h1>Name tag</h1>'
- html += tags_html
- html += ' </body>\n'
- html += '</html>\n'
- # Write HTML to a file
- # Compute a file name
- file_name = compute_file_name(first_name, last_name)
- with open('./' + file_name, 'w') as name_tags_file:
- # Writing data to a file
- name_tags_file.write(html)
- print('File ' + file_name + ' written.')
You can download this one, too.
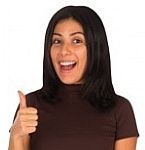
Adela
Nice!
The function compute_file_name
makes a file name in a normal form, right?
Aye. A standard format that works well on web servers.
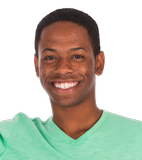
Ethan
Is this how things like Moodle work? Programs write HTML from data they have?
That's right! The data comes from a database, rather than user input, but that's the idea.
It's also how this site works. The webpage you're reading now doesn't exist, not exactly. The content is in a field in a database table. A program reads the content, and puts it in a template with the menus, book contents, exercise due dates, etc. Because you're logged in, it customizes stuff like the exercise list, to show data applying to you. It also decides which emoticon to use to show your progress.
Moodle and this site don't use Python. They use PHP, a language designed for webapps. But just like Python, PHP has variables (strings, booleans, etc.), if
statements, while
s, for
s, functions, the whole works.
You can write webapps in Python, too. I've done that in the past, and it works well.
There's sooooo much geeky fun to be had!