Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Write the missing function.
- import math
- ??????
- h = length_hypotenuse(3, 4)
- print(h)
You can use math.sqrt()
to find the square root. Feel free to test your code in Spyder before you paste it here.
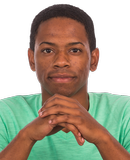
Ethan
Let's see. I know:
- The name of the function
- The number of parameters it takes
- That there's a return value.
Here's what I have so far.
- def length_hypotenuse(a, b):
- Something
- return c
Let me look up the hypotenuse formula, just to check.
Hypotenuse formula
OK. I have the sqrt
hint. So...
- c = math.sqrt(something)
Now for the middle bit.
- c = math.sqrt(a*a + b*b)
Here's the complete thing.
- import math
- def length_hypotenuse(a, b):
- c = math.sqrt(a*a + b*b)
- return c
- h = length_hypotenuse(3, 4)
- print(h)
Write the function evaluate_pokemon
for this program:
- def normalize(text):
- text = text.lower().strip()
- return text
- pokemon = input('Pokemon name? ')
- evaluation = evaluate_pokemon(pokemon)
- print('Pokemon:', pokemon)
- print('Evaluation:', evaluation)
Use these evals:
Pokemon | Eval |
---|---|
Snorlax | That's the best one! |
Paras | Paras sucks! |
Swoobat | Yuck! |
Anything else | OK |
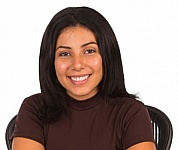
Adela
Let's see. Line 6...
- evaluation = evaluate_pokemon(pokemon)
... calls the function. It takes one parameter. To start:
- def evaluate_pokemon(pokemon):
Line 6 puts the return value into a variable, so:
- def evaluate_pokemon(pokemon):
- Something
- return evaluation
OK... Oh! There's normalize
, so I'll call that.
- def evaluate_pokemon(pokemon):
- pokemon = normalize(pokemon)
- Something
- return evaluation
Let's see... there's an eval for Snorlax, so maybe:
- def evaluate_pokemon(pokemon):
- pokemon = normalize(pokemon)
- if pokemon == 'Snorlax':
- result = "That's the best one!"
- return evaluation
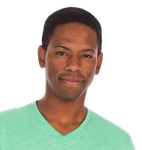
Ethan
Hey, Adela! normalize
makes everything lowercase, so...
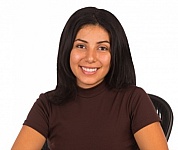
Adela
Oh, right! Test for snorlax
, not Snorlax
.
- def evaluate_pokemon(pokemon):
- pokemon = normalize(pokemon)
- if pokemon == 'snorlax':
- result = "That's the best one!"
- return evaluation
Thanks!
Now... test for the others...
- def evaluate_pokemon(pokemon):
- pokemon = normalize(pokemon)
- if pokemon == 'snorlax':
- result = "That's the best one!"
- elif pokemon == 'paras':
- result = 'Paras sucks!'
- elif pokemon == 'swoobat':
- result = 'Yuck!'
- elseif pokemon == anything else:
- result = 'OK'
- return result
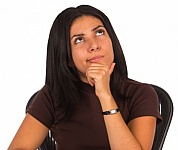
Adela
I'm not sure how to test for anything else
...
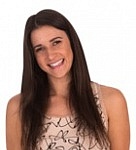
Georgina
Maybe use else
?
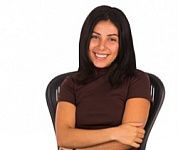
Adela
Oh! OK.
- def evaluate_pokemon(pokemon):
- pokemon = normalize(pokemon)
- if pokemon == 'snorlax':
- result = "That's the best one!"
- elif pokemon == 'paras':
- result = 'Paras sucks!'
- elif pokemon == 'swoobat':
- result = 'Yuck!'
- else:
- result = 'OK'
- return result
Yay!
Exercise
XP
You're a dungeon master. Write a program to compute the XP awarded to players for monsters they've killed. Include this code exactly:
- kali_score = compute_xp(4, 1, 5)
- vijay_score = compute_xp(3, 1, 4)
- mary_beth_score = compute_xp(2, 4, 3)
The first param is the number of orcs killed. They're worth 10 points each.
The second param is the number of goblins killed. They're worth 20 points each.
The third param is the number of giant rats killed. They're worth 7 points each.
If the number of goblins a player kills is at least two more than the number of orcs killed, add 30 XP.
Here's the output your program should produce.
- Encounter XP
- ========= ==
- Kali: 95
- Vijay: 78
- Mary Beth: 151
- Party total: 324
Upload a zip of your project folder. The usual coding standards apply.