Emily's boat pups
Not graded. So why do it?
The task
Let's write a program to make name tags. These things:
Here's the I/O (input/output) of the program:
- What is your first name? Kieran
- What is your last name? Mathieson
- Hello!
- My name is
- Kieran Mathieson
-
When you run the program, it asks for your first name (in the console pane). You type it in and press Enter. Then your last name. Type and Enter. The program will output the text of a name tag.
First try
Make a new folder and project for this example (you saw the process earlier). I called my folder name tag py
, but you can call it what you want.
Remember!
Make a new folder for every task.
Make a new Python file, with a .py
extension. I called mine name tag.py
. Call it what you want, as long as the extension is .py
.
Copy and paste this code. It has bugs we'll need to fix.
- first_name = input('What is your first name? ')
- last_name = inpt('What is your last name? ')
- full_name = first_name + last_name
- print('Hello!')
- print('My name is')
- print(full_name)
Save early, save often
Ctrl/Cmd+S. Learn that shortcut. Use it a lot.
Spyder shows something's wrong.
How is Spyder showing you something is wrong?
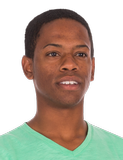
Ethan
It has some red marks in the editor.
Right. When you see those, you have something to fix.
Run the program. F5, Run in the menu, or the run button:
Type in your first name. Here's what I got in the console.
What is the mistake? What line is it in?
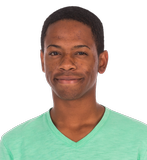
Ethan
You mistyped input
as inpt
, in line 2. Python doesn't know what that means.
Right!
Python won't automatically change inpt
to input
, but it will tell you about the issue.
This type of error is a syntax error. A word Python doesn't recognize. These errors are usually easy to find and fix. Spyder is good at showing them to you.
OK, fix the problem in the code. You'll see Spyder's error markers go away.
Save the file. Run the program. Type your names in the console.
Here's what I got:
- What is your first name? Kieran
- What is your last name? Mathieson
- Hello!
- My name is
- KieranMathieson
input
and spacing
Here's a tiny little detail, but it annoys some people. Some code:
- best_dog = input('Who is the best dog?')
- best_goat = input('Who is the best goat? ')
Here's what I got when I ran it, with some stuff I typed.
- Who is the best dog?Bert
- Who is the best goat? William
What's the difference between the two input lines the user sees?
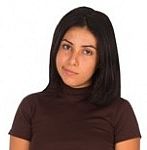
Adela
Not much. There's a space after the ? in the second line, but not the first.
Which one do you like better?
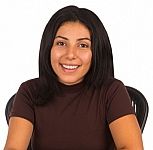
Adela
I like the second one better. Easier to see where the user started typing.
Aye, I like that one better too.
When you use input
, try to remember to add a space at the end of the prompt.
- best_dog = input('Who is the best dog? ') ← This
- best_dog = input('Who is the best dog?') ← Not this
It's a tiny thing, but users will sometimes judge your work over details like this. It's not right, but that's what happens.
Matt's Ella. A dog, not a horse.
Another problem
Hmm. No error messages, but the output isn't quite right.
What's the problem?
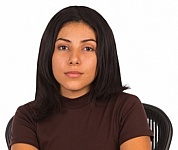
Adela
There should be a space between the first and last names in the output, but there isn't.
Aye, that's it.
This is a logic error. The program runs and produces output, but it's not the output we want.
That's how you find logic errors: compare what the program actually does with what you want it to do.
In the next lesson, we'll see how the program works, and fix the error.
Running code in the console
Up to now, we've used the console for I/O, but you can put code into it directly. It's a good way to test something simple.
In the console, type 2 + 3
, and press Enter.
What happened?
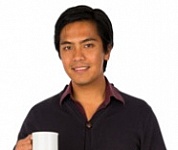
Ray
It worked out whatever I typed in.
Right! We'll run code in the console now and again.
Summary
Read summaries, and facts from the page will be stickier in your memory.
- Make a new project and folder for each task.
- A syntax error is something Python doesn't recognize. Spyder usually marks them for you.
- A logic error is when the program runs and produces output, but it's not the output we want.
- You can run code in the console.
- Send me photos of your pets.