An expression is a calculation of some sort. An example you've seen:
- first_name + ' ' + last_name
This expression has three types of thing in it:
- Variables:
first_name
andlast_name
. Each variable is a piece of memory with some data in it. - A constant: the
' '
. That's a fixed value that will never change.' '
will always be one space, no matter what else is going on. - Operators: the
+
s. Operators combine variables and constants. The first+
combinesfirst_name
and' '
. The second+
combines the result of the first one with whatever valuelast_name
has.
Your turn.
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Here's a line of Python:
- lyric = "I'm just a poor boy, from a poor family."
Why does the string constant "I'm just a poor boy, from a poor family." have double quotes?
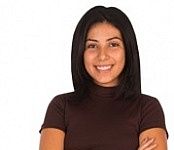
Adela
The string has I'm
in it. Would the quote in that confuse Python, if you were using single quotes?
Right! Try running this program:
- lyric = 'I'm just a poor boy, from a poor family.'
- print('The lyric is: ', lyric)
-
What happened?
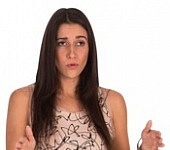
Georgina
A syntax error. The quote confused Python, like Adela said.
Computers really are stupid.
Aye, they are.