Reminder
A while loop has the form:
- while condition:
- action
condition
is either true or false, just like an if
uses. Python will keep doing action
while condition
is true.
We saw loops using string variables in the condition:
- best_pet = ''
- while best_pet != 'dog':
- best_pet = input('What is the best pet? ');
- print('Best pet: ', best_pet)
Counting
We can also use numbers in loop conditions. For example:
- count = 0
- message = ''
- while count < 3:
- message += 'doggos '
- count += 1
- print(message)
What's the output?
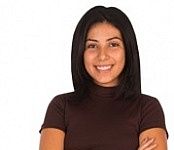
Adela
I got doggo three times: doggo doggo doggo
Right. So the program sticks together a word (doggo) the number of times in the while
.
Remember
Doggos are the best things ever!
Let's track how it works with the debugger. Start the debugger, and it will stop before running line 1. Run the next coupla lines, so it stops on line 3. We're about to run the loop for the first time.
What happens next?
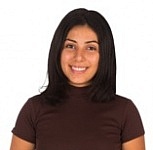
Adela
Python checks count
. It's less than three, so Python goes to line 4.
Right!
You can check expressions in the console. Recall, an expression is some calculation.
- Numeric expression: 2 + 3 * 4, evaluates to a number
- String expression: 'Doggos' + ' ' + 'rock!', evaluates to 'Doggos rock!'
- Logical expressions:
count < 3
, evaluates toTrue
orFalse
You can type expressions in the console, hit Enter, and see the result. For example:
The debugger stopped before running line 3. I typed (actually, copy-and-pasted) a logical expression in the console, and it told me its value.
Now I'm gonna run the next three lines, 3, 4, and 5. Press Ctrl+F10 three times... there.
Because of line 4, Python slaps 'doggos ' on the end of message
. The variable was MT, so now it's 'doggos '.
+=
is "add to."
Line 5 adds one to count
.
What next?
After you run line 5, what's the next line Python will run?
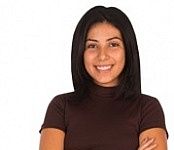
Adela
Back to line 3, to continue the loop.
Right. We've run through the loop once. You can see that in count
.
What's the value of count < 3
at this point? What line will run next?
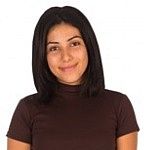
Adela
count < 3
is still True, so do the loop's code block again, starting at line 4.
Correct!
Run lines 4 and 5, then back to the while
.
count < 3
is still True, so run the loop's block again.
Add to doggos
, add to count
, then back to line 3:
This time, count < 3
is False.
What's the next line to run?
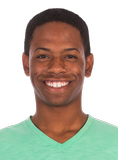
Ethan
The logical expression count < 3
is False, so skip the while
's code block. Next line to run would be line 6.
That's right. Output message
, and end.
A new data type
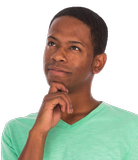
Ethan
I noticed something strange when running the code:
What's that about?
Ah! Good eye for detail.
We've seen two data types so far: float for numbers, and string for text (actually, anything we can type). Now there's a third.
An integer is a whole number, like 7 and -13, but not 9.12 or -4.1.
Integers are primarily used for counting things. For example, if you're counting people, you have 23 people or 24 people, not 23.4 people. I wouldn't want 0.4 of a person at a party. Eeewwww.
Python uses integers where it can. So with the code...
- count = 0
... Python sees the whole number (the zero), and makes count
an integer.
Generally:
- Use integers for counts
- Use floats for other things
Adding
Another program:
- total = 0
- count = 0
- while count < 5:
- new_value = float(input('Value? '))
- total += new_value
- count += 1
- print('Total: ' + str(total))
What does the program do? If you don't know, try it.
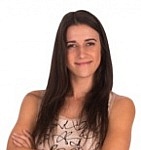
Georgina
It asks you for 5 numbers, then tells you their total.
Right!
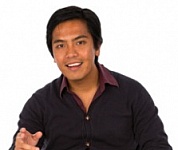
Ray
Hey... these lines:
- count = 0
- while count < 5:
- ...
- count += 1
It looks to me count
is what tracks how many times the loop is run, is that right?
Right. You could call it a loop control variable.
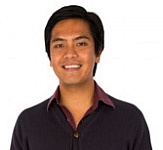
Ray
OK, so, count
starts at 0, goes to 1 first time through the loop, then 2, then 3, then 4, then... No, 4 is the last value, because it loops while count < 5
.
The program runs these lines 5 times:
- new_value = float(input('Value? '))
- total += new_value
The first one asks the user to type a number, the second one adds it to total
. That sequence gets run 5 times.
So, the program adds up 5 numbers the user types.
Good! That's exactly what it does.
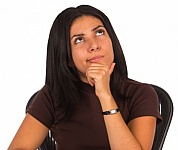
Adela
Wait, the program we had before, with the doggos, some of it is the same.
Program A | Program B |
---|---|
|
|
Right. They make a common pattern.
What's a pattern?
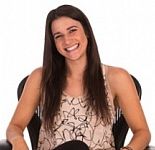
Georgina
It's a common way of doing things, people find useful.
Yes, that's it.
Once you know a pattern, you can use it to write hundreds of different programs. When you're doing a task, don't start by asking yourself,
"Self, what Python statements do I use?"
Instead...
"Self, what patterns do I use?"
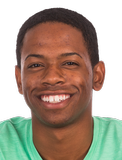
Ethan
Do these patterns work with other languages, or just Python?
Ooo, good question! They work with other languages, too. Here are versions of the totalling program in different languages.
Python | JavaScript | C# |
---|---|---|
|
|
|
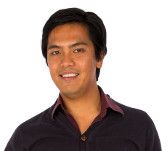
Ray
Wait. So when we're learning Python, we're learning other languages too? Kinda?
Yes. The first language is the hardest. The rest are easier because you already know some of the logic those languages use.
Summary
- Loops can use numeric control variables (like
while count < 5:
), as well as strings (likewhile response != 'dog':
). - A new data type: integer. Integers are whole numbers.
- Learn one programming language, and others are easier to learn.