Define functions before you use them
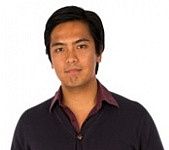
Ray
I tried this, and got the error NameError: name 'rect_area' is not defined
.
- print('The area is ' + str(rect_area(5, 6)))
- def rect_area(width, length):
- return width * length
Oh, right. Python runs code from top to bottom. When it tries to run the first line, it doesn't know what rect_area
is yet.
Put the function above the code using it, and it should be fine.
- def rect_area(width, length):
- return width * length
- print('The area is ' + str(rect_area(5, 6)))
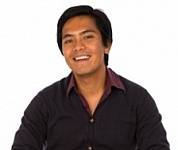
Ray
Hey, that worked!
Comments
You should add comments to functions to explain what they do. There's a special way to do this, and Spyder can help.
Add a blank line after the function def
:
Now type ''' - that's three single quotes:
Spyder shows you a suggestion in blue. Hit Enter. Spyder checks out the function's signature, and creates a template for you to fill out.
You don't have to use the Spyder tool. It's just text that you can type.
Type in what the function does, the parameters, and what the function returns.
That's called a docstring, and it's a standard way to document a Python function.
Here's something cool. Put the cursor in of just after a call to your function, and press Ctrl+I. You'll see a formatted version of your docstring in the Help panel.
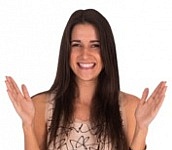
Georgina
That's so nice!
You could make a library of functions, and share it with others. They could see what each function does, right in Spyder.
Aye! That's precisely what people do.
There are programs that will scan your code looking for docstrings, and make webpages out of them. Upload them to a server, and you have online docs for your code.
From now on, you should make docstrings for your functions.
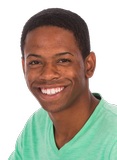
Ethan
We've been using # to make comments. Is the three quotes thing related?
Yes. You can use ''' to make any multiline comments you want. These are the same:
- # This is a multiline comment.
- # about how nice doggos are.
- # Doggos are the best things ever!
- '''
- This is a multiline comment.
- about how nice doggos are.
- Doggos are the best things ever!
- '''
Industry practice is to use ''' for docstrings, but you can use them for anything you want.
Return multiple values
Sometimes you want to return more than one value from a function. For example, here's a tip program.
- meal_cost = float(input('Meal cost? '))
- tip = meal_cost * 0.15
- total = meal_cost + tip
- print('Tip: '+ str(tip) + ' Total: '+ str(total))
The program takes one input (meal cost) and computes two values (tip and total). Let's make a function for computing the tip. Problem is, we want two values from the function, but the return
s we've seen so far have returned only one.
In Python, you can return multiple values from a function, like this:
- def compute_tip(meal_cost):
- tip = meal_cost * 0.15
- total = meal_cost + tip
- return tip, total
- meal_cost = float(input('Meal cost? '))
- tip, total = compute_tip(meal_cost)
- print('Tip: '+ str(tip) + ' Total: '+ str(total))
Line 7 calls the function. It returns two values (line 4), and are put into the variables in line 6.
(OK, yes, maybe it's one return value that's a tuple, but we don't care. It's returning multiple values.).
Finish this program. Try your solution in Spyder before you paste it here.
- radius = float(input('Radius? '))
- diameter, circumference, area = circle_stats(radius)
- print('Radius: ' + str(radius))
- print('Diameter: ' + str(diameter))
- print('Circumference: ' + str(circumference))
- print('Area: ' + str(area))
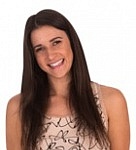
Georgina
Let's see. The function circle_stats
is missing. I'll write the first line, based on the call in line 2.
- def circle_stats(radius):
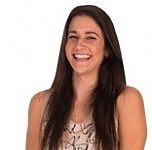
Georgina
The return
sends back three values.
- def circle_stats(radius):
- Stuff
- return diameter, circumference, area
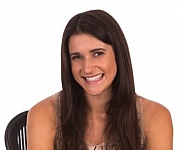
Georgina
Here's the rest.
- def circle_stats(radius):
- diameter = radius * 2
- circumference = diameter * 3.14159
- area = 3.14159 * radius * radius
- return diameter, circumference, area
- radius = float(input('Radius? '))
- diameter, circumference, area = circle_stats(radius)
- print('Radius: ' + str(radius))
- print('Diameter: ' + str(diameter))
- print('Circumference: ' + str(circumference))
- print('Area: ' + str(area))
Yay!
Summary
- Define functions before you use them.
- Use docstrings to document Python functions.
- You can return more than one value from a function.
Exercise
Goat bling
From WikiMedia
A group of your goatty friends are going to New Orleans for Mardi Gras. They want to take some bling, but are not sure how much they need. Write a program to help out.
There are big goats and small goats. Here are bling recommendations.
- Sequins: 0.4 kilos for bigs, 0.15 for smalls.
- Glitter: 0.2 kilos for bigs, 0.1 for smalls.
- Bead strands: 1.2 kilos for bigs, 0.7 kilos for smalls.
- Neck clocks (like Flavor Flav wears): two for bigs, one for smalls.
Users input number of bigs and smalls, and your program recommends bling to take.
Sample I/O:
- Bling estimator
- ===== =========
- Big goats? 1
- Small goats? 1
- You should take:
- Sequins: 0.55 kilos
- Glitter: 0.3 kilos
- Bead strands: 1.9 kilos
- Clocks: 3
More:
- Bling estimator
- ===== =========
- Big goats? 33
- Sorry, please enter a number more than zero, and ten or less.
- Big goats? 3
- Small goats? some
- Sorry, you must enter a whole number.
- Small goats? 5
- You should take:
- Sequins: 1.95 kilos
- Glitter: 1.1 kilos
- Bead strands: 7.1 kilos
- Clocks: 11
For the inputs, keep asking until you get a valid number.
Write an input function with this docstring:
- '''
- Input a goat count.
- Parameters
- - - - - -
- prompt : string
- Question to ask.
- Returns
- - - - -
- value : int
- Count.
- '''
Write a computation function with this docstring:
- '''
- Compute the amount of bling a goat group will need.
- Parameters
- - - - - -
- bigs : int
- Number of big goats.
- smalls : int
- Number of small goats.
- Returns
- - - - -
- sequins : float
- Kilos of sequins.
- glitter : float
- Kilos of glitter.
- bead_strands : float
- Kilos of bead strands.
- clocks : int
- Number of neck clocks.
- '''
Upload a zip of your project folder. The usual coding standards apply.