We're going to add validation loops to the tip program. It's a topic that confuses some people.
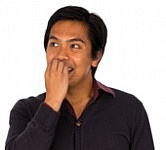
Ray
Uh oh.
Don't worry, we'll take it easy. Let's start with a simple task.
Just the dogs
Let's write a program asking users for the best pet. It keeps asking until they type the right pet, which is, of course, dogs.
Here's sample I/O:
- What is the best pet? cat
- What is the best pet? llama
- What is the best pet? lizard
- What is the best pet? dog
- Best pet: dog
The program keeps asking until you give it the right answer.
My friend Burt
Getting started
Here's one way to write the program:
- best_pet = ''
- while best_pet != 'dog':
- best_pet = input('What is the best pet? ');
- print('Best pet: ', best_pet)
Line 1 creates an MT string variable.
Line 2 starts a while loop. It has the form:
- while condition:
- action
condition
is either true or false, just like an if
uses. Python will keep doing action
while condition
is true.
What's the !=
in line 2 mean?
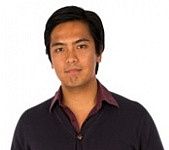
Ray
Not equal to, right?
Yes.
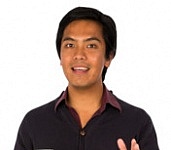
Ray
Hey. In the code...
- best_pet = ''
- while best_pet != 'dog':
- best_pet = input('What is the best pet? ');
- print('Best pet: ', best_pet)
The first line, best_pet = ''
, why is that there?
Why is line 1 there?
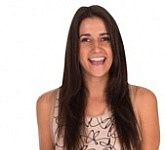
Georgina
Ooo! I know! It's so line 2 works the first time.
If you try while best_pet != 'dog':
but there is no best_pet
variable, the program will crash.
So, make best_pet
, and make it MT.
Aye! That's right.
Comments
Hey, Adela, can you add some comments to the code?
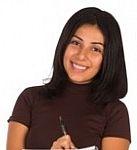
Adela
OK.
- # Initialize best_pet to MT.
- best_pet = ''
- # While best_pet does not equal dog...
- while best_pet != 'dog':
- # Ask the user what the best pet is.
- best_pet = input('What is the best pet? ');
- # Output the best pet.
- print('Best pet: ', best_pet)
Good! Thanks, Adela. We can see the purpose of each bit of code now.
Adela added one comment per line of code. That's not normally what you do. You add a comment for each chunk, a chunk being a sentence you would say when explaining the code to someone else. Like, "that's the input," and "that's processing."
Here, the chunks are so simple that each is just one line of code.
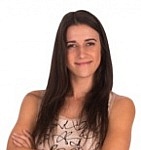
Georgina
That's not a very precise way of defining a chunk.
True. English is not a precise language. Write comments so that, if you just read the comments, you would understand how the code works.
Clean up user input
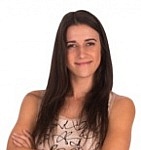
Georgina
What if they type "Dog" instead of "dog"?
Oh, good point. Or they might type DOG or doG or add some spaces.
Fill this in:
When we __________________ a variable, we convert it to a common format that's easier to test.
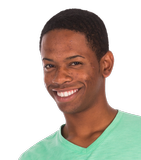
Ethan
Normalize, right?
Aye. Georgina, how would you normalize user input?
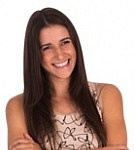
Georgina
Take the user input, strip excess spaces, and make it lowercase. Like we did before.
OK, let me see... we had:
- service_level = input('Service level (g=great, o=ok, s=sucked)? ')
- service_level = service_level.strip().lower()
Normalization is on the second line, removing extra spaces, and making everything lowercase. So...
- # Initialize best_pet to MT.
- best_pet = ''
- # While best_pet does not equal dog...
- while best_pet != 'dog':
- # Ask the user what the best pet is.
- best_pet = input('What is the best pet? ');
- # Normalize
- best_pet = best_pet.strip().lower()
- # Output the best pet.
- print('Best pet: ', best_pet)
Try the program. Does it work?
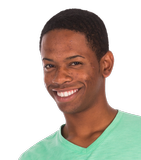
Ethan
Yes, it does.
To infinity, and beyond!
Here's some Evil Code:
- # Initialize best_pet to MT.
- best_pet = ''
- # While best_pet does not equal dog...
- while best_pet != 'Dog':
- # Ask the user what the best pet is.
- best_pet = input('What is the best pet? ');
- # Normalize
- best_pet = best_pet.strip().lower()
- # Output the best pet.
- print('Best pet: ', best_pet)
It's the same as before, except I changed one letter.
Hey Georgina! Can you try running it?
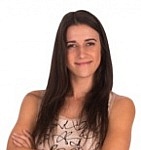
Georgina
OK.
- What is the best pet? bird
- What is the best pet? cat
- What is the best pet? dog
- What is the best pet? Dog
- What is the best pet? doggo
- What is the best pet?
- What is the best pet? stop
- What is the best pet? help!
- What is the best pet?
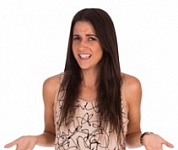
Georgina
What... It won't stop when I type dog
. Or anything else, it looks like.
You said you changed one letter...
Oh, you changed...
- while best_pet != 'dog':
... to...
- while best_pet != 'Dog':
Oh. I see it now.
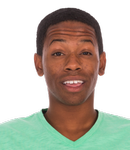
Ethan
See what?
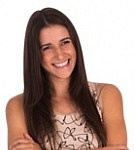
Georgina
Nice trick, Kieran!
- # Initialize best_pet to MT.
- best_pet = ''
- # While best_pet does not equal dog...
- while best_pet != 'Dog':
- # Ask the user what the best pet is.
- best_pet = input('What is the best pet? ');
- # Normalize
- best_pet = best_pet.strip().lower()
- # Output the best pet.
- print('Best pet: ', best_pet)
Line 8 normalizes best_pet
. It will always be lowercase. Like, Dog
becomes dog
.
On line 4, best_pet
will always be not equal to Dog
. There will never be an uppercase letter in best_pet
! The test in the while
will always be true, so it keeps looping forever.
Good! You worked it out.
This is an infinite loop. It will keep running around and around, no matter what the user types. The infinite loop is a common bug.
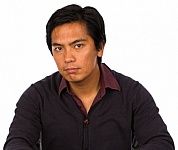
Ray
Umm... how do we stop the program? Reboot?
That'll work, but there's an easier way. Ctrl+C.
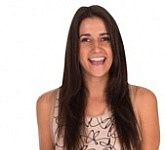
Georgina
Let me try it...
- What is the best pet? doggo
- What is the best pet?
- What is the best pet? stop
- What is the best pet? help!
- What is the best pet? Ctrl+C pressed here Traceback (most recent call last):
- ...
- KeyboardInterrupt: Interrupted by user
Good. If... no, when... you get stuck in an infinite loop, Ctrl+C will stop the program.
OK, that's the basics of a while
loop. While a test is true, keep doing some code.
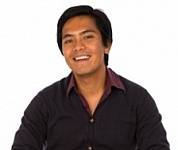
Ray
Wait, that's it? This is a short lesson.
Best to learn in small pieces. Each piece should be easy to understand.
Summary
while
s have a condition and action. Python will keep doing the action while the condition is true.- Variables used in the condition, like
doggos
inwhile doggos < 2:
, should exist before thewhile
is run for the first time. - Break infinite loops with Ctrl+C.