In the last lesson, the Scoobies wrote the input part of a "Goats in Space-ace-ace..." program. There were three input variables: small_goats
, medium_goats
, and large_goats
. The validation loops for each one were almost the same. Here's the small goat code, with the lines that change for medium and large goats marked.
- # How many small goats?
- is_input_ok = False
- while not is_input_ok:
- # Start off assuming data is OK.
- is_input_ok = True
- # Get input from the user.
- user_input = input('Small goats? ')
- # Is the input numeric?
- try:
- small_goats = int(user_input)
- except ValueError:
- print('Sorry, you must enter a whole number.')
- is_input_ok = False
- # Check the range.
- if is_input_ok:
- if small_goats < 0 or small_goats > 100:
- print('Sorry, please enter a number zero or more, but no more than 100.')
- is_input_ok = False
(I didn't include the testing code.)
There are about 670 characters here. Only 35 characters are specific to small goats. That's only 5%. When we copy the code for medium and large goats, 95% of the code remains the same.
Why is all this repeated code a problem?
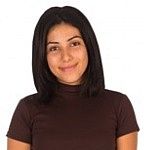
Adela
I can think of a few things, but I'm guessing the most important is that updating the code would cost more.
Right! If we need to change something, we need to change it three times, then test each one. Easy to make a mistake.
Goat validation function
OK, let's use this stuff on our goat problem. Here's the code again. The highlighted stuff is what we want to be different for medium and large goats.
- # How many small goats?
- is_input_ok = False
- while not is_input_ok:
- # Start off assuming data is OK.
- is_input_ok = True
- # Get input from the user.
- user_input = input('Small goats? ')
- # Is the input numeric?
- try:
- small_goats = int(user_input)
- except ValueError:
- print('Sorry, you must enter a whole number.')
- is_input_ok = False
- # Check the range.
- if is_input_ok:
- if small_goats < 0 or small_goats > 100:
- print('Sorry, please enter a number zero or more, but no more than 100.')
- is_input_ok = False
You could make a function out of that, reusing it for every goat type.
- def input_num_of_goats(prompt):
- is_input_ok = False
- while not is_input_ok:
- # Start off assuming data is OK.
- is_input_ok = True
- # Get input from the user.
- user_input = input(prompt)
- # Is the input numeric?
- try:
- goats = int(user_input)
- except ValueError:
- print('Sorry, you must enter a whole number.')
- is_input_ok = False
- # Check the range.
- if is_input_ok:
- if goats < 0 or goats > 100:
- print('Sorry, please enter a number zero or more, but no more than 100.')
- is_input_ok = False
- return goats
- small_goats = input_num_of_goats('Small goats?')
- medium_goats = input_num_of_goats('Medium goats?')
- large_goats = input_num_of_goats('Large goats?')