Not graded. So why do it?
Not graded. So why do it?
Can't open the hood
Your new car
Your brand-new car! That's soooo cool! Groovy, rad, whatever else old people say.
There's a strange noise from the engine. Ker-chunk-ker-chunk. Doesn't sound good.
You take it to a mechanic. They say, "Pop the hood." You can't find the button to open it. The mechanic can't find it either. Turns out, the engine compartment is welded shut! ARGH!
"If I can't look inside, I can't tell what's wrong," says the mechanic, quite reasonably.
Complex machines have many hidden parts. If the machine isn't working right, you often need to look at the hidden bits to work out what's wrong. If you can't see the hidden bits, figuring out what's wrong is a lot harder.
Same for people. X-ray and MRI machines are look-insiders, so we can work out why joints and other things aren't working right.
Same for programs. You can't see inside them, normally. When they don't work right, you can't tell why.
Fortunately, there are look-insiders for programs, called debuggers. They won't actually fix bugs. They let you look inside a program, and work out where evil bugs are. Then you know what code to fix.
Debug mode
Here's the code again, with the missing-space bug.
- first_name = input('What is your first name? ')
- last_name = input('What is your last name? ')
- full_name = first_name + last_name
- print('Hello!')
- print('My name is')
- print(full_name)
The program puts user input in variables. It works out another variable. Then it outputs string constants, and the variable it worked out.
But the output isn't right.
Let's run the program in debug mode, so we can look inside. Here's the button:
Give it a click (or Ctrl+F5). You'll get:
In debug mode, Spyder can run one line of code at a time. It highlights the line that's about to run with an arrow. It hasn't run line 1 yet.
The line that Spyder's about to run (when we tell it to) is:
first_name = input('What is your first name? ')
What is Python going to do when it runs this line?
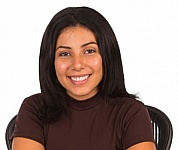
Adela
It's gonna show the prompt - What is your first name? - in the console. Then wait for you to type something and press Enter.
Let's see if you're right. Use the run-current-line button (Ctrl+F10):
Look in the console, and you'll see a question:
What is your first name?
Click in the console, type your name, and press Enter.
Yay! Adela was right! This small mammal is happy.
Variables are invisible
Variables are hidden inside the computer. They don't show up anywhere on the screen. Makes it hard to see what the program is doing.
Fortunately, a tool called the Variable Explorer shows variables as the program is running.
I'll open the Variable Explorer's tab:
At this point, Spyder has run the first line of the program, and I typed something in. It has stopped on the second line, waiting. This is what I see:
The Variable Explorer shows variables that have been made, and their values. There's only one variable so far, first_name
. It has Kieran
in it, or whatever you typed. It takes six characters of memory.
The type of the variable is str
, for string. A variable's type tells you what sort of data the variable can contain. A string variable holds text. More accurately, anything you can type on the keyboard.
Now I'll run line 2, with Ctrl+F10 (or click the run-next-line button). Here's line 2:
- last_name = input('What is your last name? ')
It's almost the same as line 1. The prompt is different, and it puts data into a different variable. Here's what I got:
Now we have two variables.
Execute the next line, line 3, with Ctrl+F10. Here's what I got:
Spyder ran the line, and stopped on the next one. full_name
, created in line 3, shows in the Variable Explorer (VE).
We can see the mistake in full_name
. Spaceless. We now know where the problem is, though the debugger doesn't tell us how to fix it. Fortunately, you guys figured that out in the last lesson.
This is what debugging is about.
Debugging
Use a debugger to compare:
- The variable values you wanted the program to have when you wrote the code.
- The values they actually have when the code runs.
We wanted full_name
to be Kieran Mathieson
, but we got KieranMathieson
. We can tell that by the time line 4 has run, the error has already happened. So, we can focus on the code above that point.
That's the basic idea. Compare (1) what the program is doing with (2) what you wanted it to do.
Like debugging a human's teeth. The debugger, an x-ray machine, doesn't fix anything. It shows the dentist where the problam is, so they can fix it themselves.
Remember, debuggers don't fix errors for you. They help you track down where errors are. Then you fix them yourself.
Stepless
You can step through one line at a time, but that's inconvenient if the line you suspect is evil is 93 lines down. Breakpoints help with that.
Set a breakpoint by clicking on the gray area next to a line number.
When you debug (Ctrl+F5), Spyder will still stop on the first line. Press it again, and Spyder will run your code as normal, until it gets down to the breakpoint. It will stop before running the line.
The debugger is your friend
The debugger is a look-insider. You can watch your program run line by line. You can set breakpoints.
Get into the habit of stepping through your code. It can be satisfying to see the computer following your every command.
Summary
- You can't see inside programs when they run, normally.
- Debuggers let you look inside programs as they run. You can see what lines of code will run next, and what values variables have.
- Spyder's debugger is your friend.
Exercise
Breakpoint
Here's a program:
- my_first_name = 'Kieran'
- my_last_name = 'Mathieson'
- fave_pet_name = 'Burt'
- output = my_first_name + ' ' + my_last_name + ' loves ' + fave_pet_name + '.'
- print(output)
Copy it to a new file in a new Spyder project. Change the text, using your names, and the name of your fave pet. If you don't have a fave pet, make up a name, like Gootenliky. Or something.
Put a breakpoint on the last line. Debug the program, and show VE. Take a screenshot of your program stopped on the last line, showing the variables in VE. For me, this would be:
Submit your screenshot.