A nested if
is an if
inside another if
. They're common.
An example. Some older people have trouble with..., er, their digestive processes (yet another reason old people suck). A dance club keeps prune juice for them, in a quiet room in the back.
- age = float(input('How old are you? '))
- if age >= 21:
- print('Welcome! Bring your money inside.')
- if age >= 50:
- print("There's prune juice in a quiet room in the back.")
- else:
- print("Sorry, you're not allowed in yet.")
The geezer test is inside the can-enter-club test. You can put as much code as you want inside an if
block, including other if
s. The inner if
is called a nested if
.
This lets you break conditions down into easier tests. Easy is good. First, can they enter the club at all? That's the test in line 2. Then, for those who can, are they old and useless? That's the test in line 4.
Line 5 runs if the test in line 4 is true. Of course, line 4 runs only if the test in line 2 is true.
You could also write the code without nested ifs, like this:
- age = float(input('How old are you? '))
- if age >= 21 and age < 50:
- print('Welcome! Bring your money inside.')
- elif age >= 21 and age >= 50:
- print('Welcome! Bring your money inside.')
- print("There's prune juice in a quiet room in the back.")
- else:
- print("Sorry, you're not allowed in yet.")
Why is this not as good as the nested if solution?
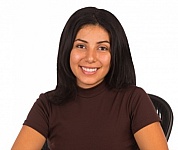
Adela
Lines 3 and 5 are repeated. If you want to change the output, you might forget, and change just one.
Right!
There's something else, too. In Australia, and many other countries, the legal drinking age is 18, not 21.
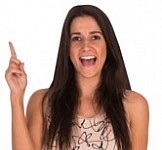
Georgina
Oh! The 21 is in two places! If you wanted to use the program in Australia, you'd need to change 21 to 18 in more than one place. Easy to forget one.
The geezer test is in two places, too. If you want to change it to, Ida know, 53, it's easy to forget one.
Good! This is the DRY principle.
Nested ifs might seem more complicated at first, but they actually make programs simpler and easier to maintain.
The debugger is your friend when writing nested if
s. Run one line at a time. See which statements your program executes.
In fact, you might want to practice with the code above. I'm just sayin'.
Summary
- You can put as much code as you want inside an
if
's code block, including otherif
s. - That's called a nested if.